Anything above the line is updated as of November 11th, 2023. Anything below the line is part of the old guide. I will eventually make an index.
Hey, uh, Dark, what the hell is CSS?
CSS stands for cascading style sheets. It's a coding language that tells a website what to look like. These documents are called "style sheets", and when you make or edit a palette, you're making a style sheet for the website.
In CSS you use selectors to define what you want to "target". A selector is usually the name of an element, for example, if you wanted to target the body of the website as a whole, you'd use "body". There are many, many types of selectors, which I'll go over before we dig into actually coding anything.
It sounds complicated but it's easy when you get the hang of it. If you've never looked at CSS before, it can be intimidating. What are all the curly brackets and semicolons for?! I'm here to answer your questions and make palettes a less rage-inducing task. If you need a quick reference for what a word means, I'll have a pinned post under this guide with a dictionary for you.
There are more selector types??
Yeah. A lot more, actually. Your selector tells the page exactly what to target. If your code isn't working, try making the selector more specific. See the post under this one (the dictionary) for a list of some common selectors.
A fun tip, you can "group" things together to make your code neater and shorter. For example, if I wanted bold text and italic text to be red, I'd use this:
b, i {
color: red;
}
That's all fine and dandy but how do I make a palette?
Let's get to the good stuff! First, you need a premium account to make custom palettes. Got one? Awesome! You're ready to start.
Head on over to Stable > Stable Activities, and click on "Manage Palettes". It's right at the bottom, just above manage club. It looks like this:
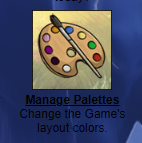
Clicking on it, you'll come to the palette page:
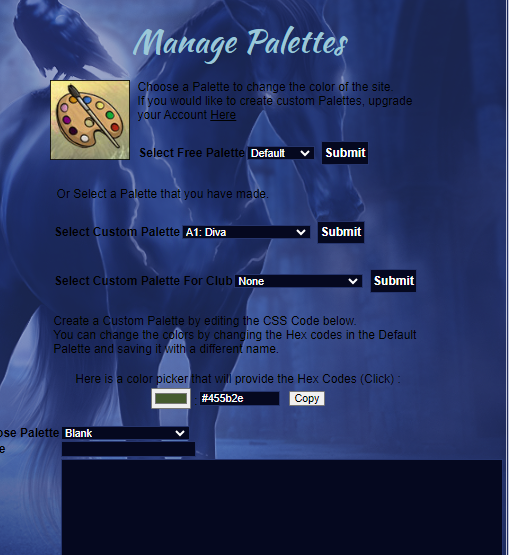
See where the dropdown says "Blank"? Click on it. Choose "Default" from the menu. Click inside of the big box with all the code in it, click control + a (command + a on a mac, this selects all the text in the box for you) and copy it all by either right clicking and choosing copy or using control/command + c. Then use that dropdown again (or refresh the page), and paste it into a blank palette. Don't forget to name it!
Now you'll have a new palette. Beside "set custom palette" choose your new palette and click submit to equip it. Now to edit it you just choose the name of your custom palette from the edit dropdown and code away!
Okay but how do I code?
Before I get into breaking down what each code piece means there's important things you need to know.
CSS uses a lot of {curly brackets} and semicolons (;). Curly brackets "contain" the declaratons that you use to style things, and you end each line with a semicolon. Like this:
a {
color: red;
background-color: blue;
}
This code will make links red with a blue highlight, by the way. See how the curly brackets contain all of your declarations and a semicolon ends each line? Do not forget either or anything beneath the missing character will not work (because technically, you didn't end the line/close the declaration).
Please check the dictionary post here (or just scroll down) to read up on what the words I'm using mean!
A word about hex codes
Hey Dark what the fuck is this???

Oh yeah those. Those are called hex codes. They're a computer-y way of defining a colour. They can have numbers 0-9, and letters a-f. F is the most and 0 is the least. Each two characters correspond to red, green, and blue respectively for a total of 6 characters. #000000 is black (none of any colour) and #ffffff is white (all of every colour).
Code breakdown time!
Okay, now you can start coding!
I will include a screenshot of the section in the basic palette template and then a breakdown of exactly what each part does/changes, and some tips for that specific line of code if I have any.
You will learn how to change these afterwards. It's important to know what these do before you code so you don't make your site unuseable.
Please note that I always use either hex codes (#123abc) or rbg codes (255, 255, 255) when I am choosing site colours. It makes it easier for me to pick a specific colour instead of one of the predefined ones. However, if you like predefined colour names instead of codes, here's a list. It also has the hex code for that colour if you'd like.
Shall we begin?
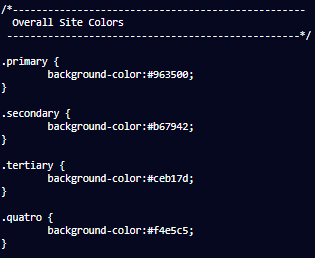
If you've been paying any attention you know the things that say ".primary", ".secondary" etc are selectors. They are no touchie. Do not change them if you want to keep your sanity. The following parts however are declarations and they can be played with!
.primary - Background colour for the chatbox container, where the text input box and post buttons appear. I usually group this and .pgbg. You'll see why soon.
.secondary - The coloured bars that hold your stable flags and the "Stable Info" text on your profile page.
.tertiary - "Stable Info", "About This Stable" etc headers on the homepage.
.quatro - Status messages and the forum main colour. For example every time you edit your palette it says "Palette has been updated." at the top. This changes those.
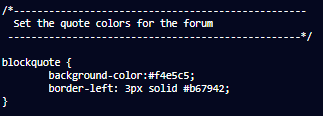
blockquote
Sets the colours for these bad boys.
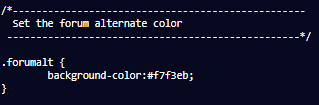
.forumalt - Sets the alternative forum colour to make the colours alternate.
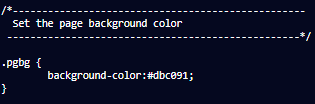
.pgbg - Sets the background colour for the main page. Brownish by default. I usually group this with .primary so that both of those parts of my page are the same colour with me only needing to change one code.
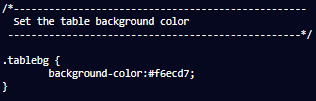
.tablebg - Sets the background colour for tables, like the light brown spot where your quests show up.
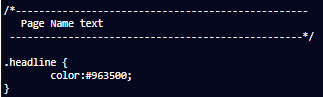
.headline - The big text that titles pages. On your stable page it says "Stable Page" at the top for example.
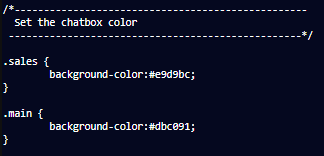
.sales and .main - Sets the background colour for chatbox posts in sales cat and main chat respectively.
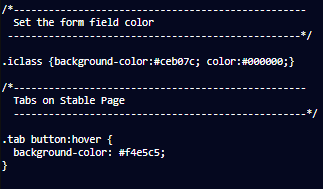
.iclass - Text input boxes. In other words, anywhere you type in text.
.tab button:hover - The hoverable tabs that say "Our Stable", "Profile" and "Games" on stable profiles. This only changes the colour when you hover over them.
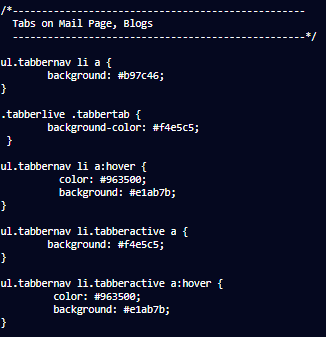
All of these change the look of the tabs on the bottom of barn and mailbox pages.
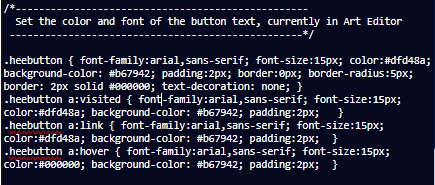
All of these change the look of text on buttons.
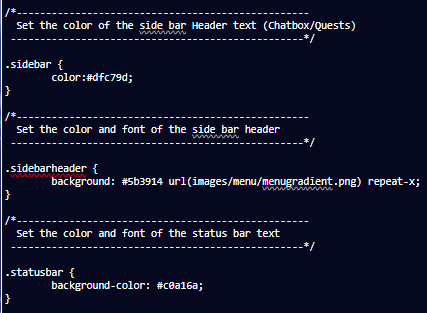
.sidebar - Changes the colour of the text that says "Chatbox" and "Quests" on the left of the screen.
.sidebarheader - Changes the font and background of the same text mentioned before this.
.statusbar - Changes the colour of your statusbar (where it shows your money and the time).
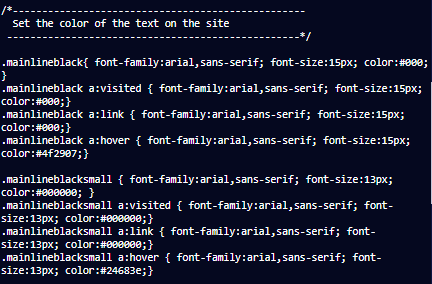
All of these change the colours of different text across the site. Anything with a suffix changes a different thing, so I've broken them down.
a:link - Changes the colour of links that you haven't visited (clicked on) before.
a:visted - Links that you've visited before. For example on most devices links are blue by default and turn purple after you've clicked on them. I usually keep this the same colour as my a:link text so the forums and chatbox links don't look weird.
a:hover - Links when you hover over them. You can add fun effects, like a text shadow, here to make your links pop out when you hover them.
Anything without "a" is just normal text, not links.
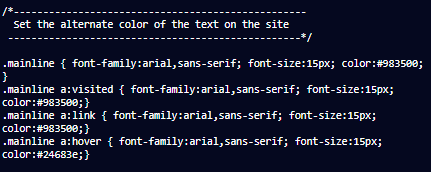
The same suffix rules apply here. These change the subtext colours instead of general text. An example is the amount of store credits you have when you look next to your avatar on your stable page.
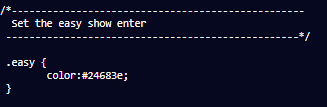
.easy - Sets the colour of the placings of horses who are competitive on the easy show enter page. Green by default.
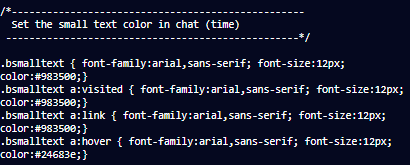
Follows the suffix rules. These change the colours of the timestamps on chatbox posts. You could group all of these together if you'd like to make your code neater.
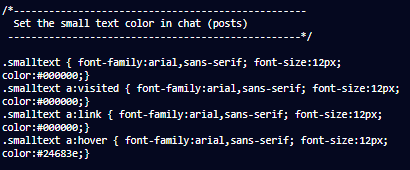
Sets the colour of post content (what people post) in the chatbox.
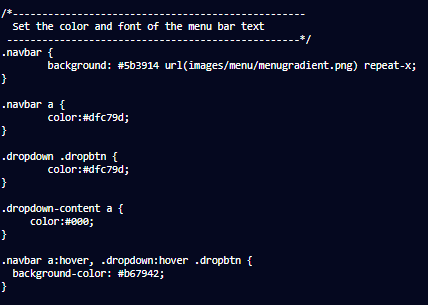
.navbar - Navigation bar as a whole. Changes the position of the navbar ("Stable", "Services", etc). Giving this a background makes all of the headers and the space between them have a background, instead of just the headers.
.navbar a - Backup option for the inner link ("Home", "Capture A Horse", etc) colours. If you don't have .dropdown-content a set, the links will use this colour instead.
.dropdown .dropbtn - Navigation bar headers ("Stable", "Services", etc).
.dropdown-content a - These are the links inside the actual dropdowns ("Home", "Capture A Horse", etc).
.navbar a:hover, .dropdown:hover, .dropbtn - Hover effects for your navbar go here.
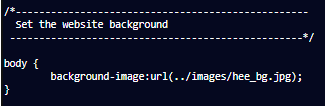
This changes the background of the website. Usually it's the picture of the trees and the field that changes with the seasons.
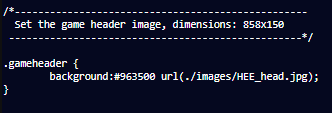
Changes the header image of the website.